How to Build Serverless Functions with Rust on AWS Lambda
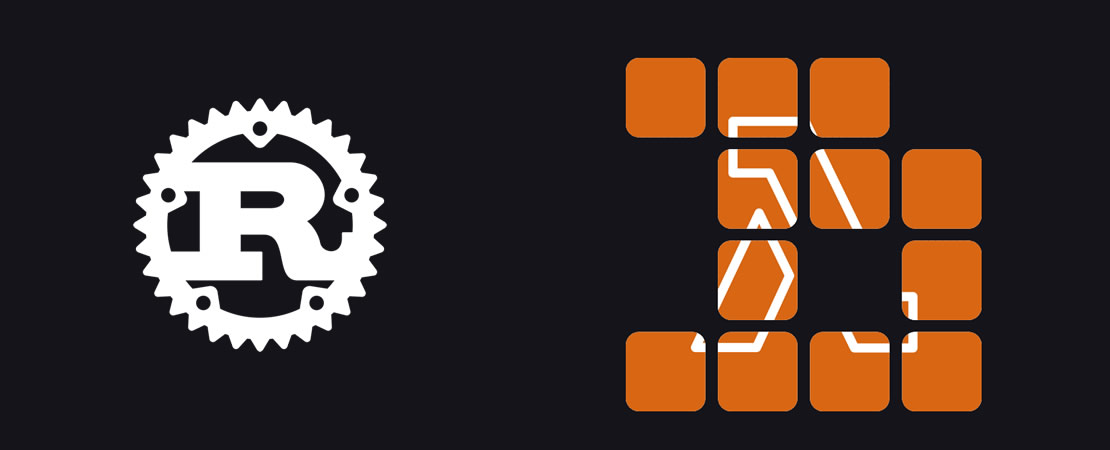
April 22, 2025
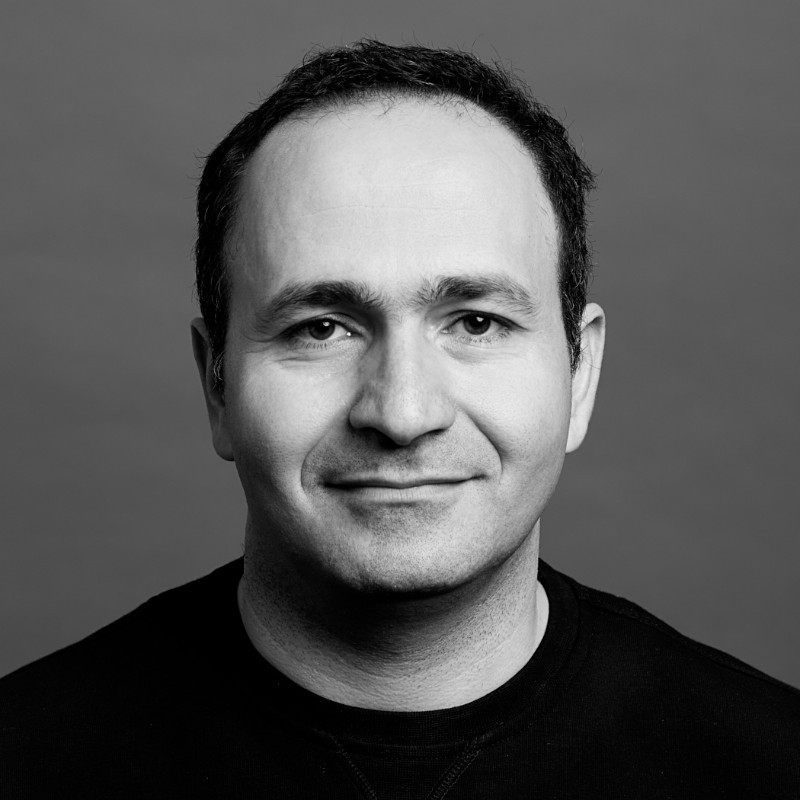
Mehdi Mohseni
From Conference Stage to Code Breakdown
This article is part of the workshop I presented at We Are Developers 2024 in Berlin, titled “Contrasting C# and Rust in AWS Lambda.”
In that session, I explored the strengths and differences between building serverless applications using C# and Rust, focusing on practical implementation in AWS Lambda environments.
Here, I will dive deeper into how to build AWS Lambda functions specifically using Rust.
Why Rust? A Language Built for Safety and Performance
Rust is a systems programming language designed with a strong focus on safety, speed, and concurrency. It guarantees memory safety without relying on a garbage collector, making it an ideal choice for performance-critical applications. Rust is built to help developers create reliable and efficient software that can scale confidently across different environments.
Originally started as a personal project by Graydon Hoare in 2006, Rust gained momentum when Mozilla began supporting its development in 2009. The language reached its first stable release, Rust 1.0, in May 2015, and since then, it has grown rapidly thanks to a strong and active global community.
Rust offers several key advantages. It delivers high performance, achieving near C/C++ level speeds without the overhead of a runtime environment. Through strict compile-time checks, Rust ensures memory safety, preventing common bugs such as null pointer dereferencing and buffer overflows. Additionally, Rust’s ownership model promotes fearless concurrency, allowing developers to write safe, multi-threaded code without the typical risks associated with concurrent programming.
Preparing the Development Environment
Rust compiles directly to native machine code, which means setting up the correct development environment is essential. Without the necessary compilers, linkers, and system libraries properly configured, you may encounter compilation errors. Here’s how to set up your environment for building Rust-based AWS Lambda functions:
1. Install WSL (Windows Subsystem for Linux)
WSL allows you to run a Linux environment on your Windows machine, enabling seamless use of Linux-based development tools. It’s an essential first step to ensuring compatibility with Rust’s toolchain and related utilities.
2. Install Rust Toolchain
You’ll need to install the Rust programming language along with its toolchain. This includes rustup
, which helps you manage Rust versions, and cargo
, Rust’s official package manager and build system.
3. Install Cargo-Lambda
Cargo-Lambda is a specialized tool for building and deploying Rust-based AWS Lambda functions. It simplifies packaging and deploying your Lambda code.
4. Install Node.js
Node.js is required for certain deployment workflows and tooling, especially when working with the AWS Cloud Development Kit (CDK) later on.
5. Install the Zig Toolchain
Zig is a dependency used to ensure cross-compilation compatibility, particularly when targeting environments like AWS Lambda, which require statically linked binaries. Installing Zig will help streamline the build process when compiling Rust code for AWS.
6. Install AWS CDK (Cloud Development Kit)
The AWS CDK allows you to define cloud infrastructure using familiar programming languages. It will be used later to deploy and manage your AWS Lambda functions and related resources.
Working with Cargo-Lambda
Cargo-Lambda is a powerful tool designed for building, testing, and deploying Rust applications as AWS Lambda functions.
It extends Cargo — Rust’s official package manager — and simplifies the creation of Lambda-compatible binaries, streamlining the entire development and deployment workflow.
Using Cargo-Lambda, you can easily scaffold new Lambda projects, build them for deployment, and even watch for file changes to automatically trigger rebuilds during development.
For quick examples and usage commands, you can refer to the official Cargo-Lambda documentation.
$ cargo lambda new new-lambda-project
$ cargo build
$ cargo lambda watch
Cargo.toml and Crates in Rust
Cargo.toml
Cargo.toml
is the configuration file for Rust’s package manager, Cargo.
It defines the metadata for a Rust project, including its name, version, and dependencies.
This file is essential for managing project dependencies, build settings, and other configurations, ensuring that the project compiles and runs correctly.
Crates
A crate is a package of Rust code.
Crates can be libraries or executables, and they help modularize and reuse code across projects.
To add a new crate, you specify it under the [dependencies]
section of your Cargo.toml
file.
For example, to add the regex
crate, you would include a dependency entry with its version.
$ cargo add [crate name]
$ cargo remove [crate name]

Dive into the code
books database stored in DynamoDB.
Using the Axum framework for routing and lambda_http for AWS Lambda integration, the service provides endpoints to:
- Fetch a book by ID
- Fetch all books
- Filter books by category
- Add a new book
- Perform a health check
AWS configurations are loaded dynamically at runtime, and Serde with serde_dynamo is used for seamless data serialization/deserialization.
Error handling and logging are powered by tracing to maintain clear observability during function execution.
Here’s a simplified view of the route setup:
This lightweight and asynchronous application is optimized for serverless environments, ensuring fast and efficient request handling inside AWS Lambda.
Compiling the Rust Project for AWS Lambda
To compile your Rust project for deployment on AWS Lambda, use the following command:
cargo lambda build --release --target x86_64-unknown-linux-musl
This command prepares the project for the AWS Lambda environment by producing an optimized, portable binary. Here’s a breakdown of each part:
cargo lambda build
: Invokes the build process using thecargo-lambda
tool, specifically targeting AWS Lambda deployments.--release
: Instructs Cargo to compile the project in release mode, optimizing the binary for better performance and smaller size.--target x86_64-unknown-linux-musl
: Specifies the build target platform.
In this case, it targets x86_64-unknown-linux-musl, which produces a statically linked, portable binary format that is fully compatible with AWS Lambda execution environments.
Building your project with these settings ensures that the compiled binary meets AWS Lambda’s requirements for deployment and executio
Deploying with AWS Cloud Development Kit (CDK)
The AWS Cloud Development Kit (AWS CDK) is an open-source software framework for defining cloud infrastructure in code and provisioning it through AWS CloudFormation.
By using CDK, developers can describe cloud resources using familiar programming languages such as TypeScript, Python, or C#, making infrastructure setup easier and more maintainable.
In this project, we use AWS CDK with TypeScript to automate the deployment of our Rust-based Lambda function.
Setting Up the CDK Deployment
First, you need to install the AWS CDK toolkit globally and initialize a new CDK application:
npm install -g aws-cdk
mkdir hello-cdk
cd hello-cdk
cdk init app --language typescript
After setting up the base CDK project, you can prepare the deployment scripts.
In the deploy.ts
file, we define a new CDK application and specify the deployment stack and environment:
Finally, to deploy the application, navigate to the deployment folder and run the following commands:
cd deploy
yarn install
yarn build
yarn cdk synth
yarn cdk deploy
This process builds the project, synthesizes the CloudFormation template, and deploys your Rust-based AWS Lambda function along with any related resources.